Twitter Bot Schedule
Twitter Bot Schedule
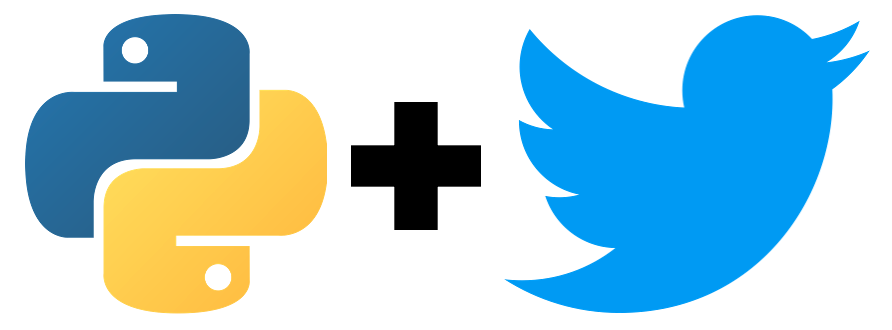
We finished out the core functionality of our Twitter Bot in our last post. But we needed a way to have our bot run and only attempt to tweet once or twice a day. If we look on how much I post which is about once a week, but I have done one off post through out the week. For my needs running once a day at a specific time should be enough. The easiest way to do this is with a library. For this we are going to use schedule a scheduling library written by Dan Bader. This is super easy to use and we are not re-inventing the wheel. To add it to our project we run the following command:
pip install schedule
Let’s now modify the bot.py
add in schedule
and time
libraries but also clean up our other from statements:
from posts import get_todays_post
from tweets import see_if_tweet_exist, tweet_latest_post
import schedule
import time
In the original write up we had our import section looking something like this:
from posts import *
from tweets import *
Yes this works, but we didn’t need everything from those files so we wanted to narrow it down to what we only need. Now we can rework the def main():
and add in a new method called def run_new_post():
. The functionality we had in def main():
we need to move to def run_new_post():
:
def main():
post = get_todays_post()
if post.link:
if not see_if_tweet_exist(post.link):
tweet_latest_post(post)
To something like this:
def main():
run_new_post()
def run_new_post():
print('start')
post = get_todays_post()
if post.link:
if not see_if_tweet_exist(post.link):
tweet_latest_post(post)
print('end')
This gives us the ability to add in schedule
to our def main():
I also added in some print()
so when this runs we can see it as an output. Now to add in schedule
:
def main():
schedule.every().day.at("09:00").do(run_new_post)
while True:
schedule.run_pending()
time.sleep(1)
This will have our app run, but it will only attempt to tweet a new post only at 9 o’clock. This was a quick and easy update to our app gives us the right structure to have it deployed and running somewhere that isn’t my laptop. I keep my work in this GitHub repo.